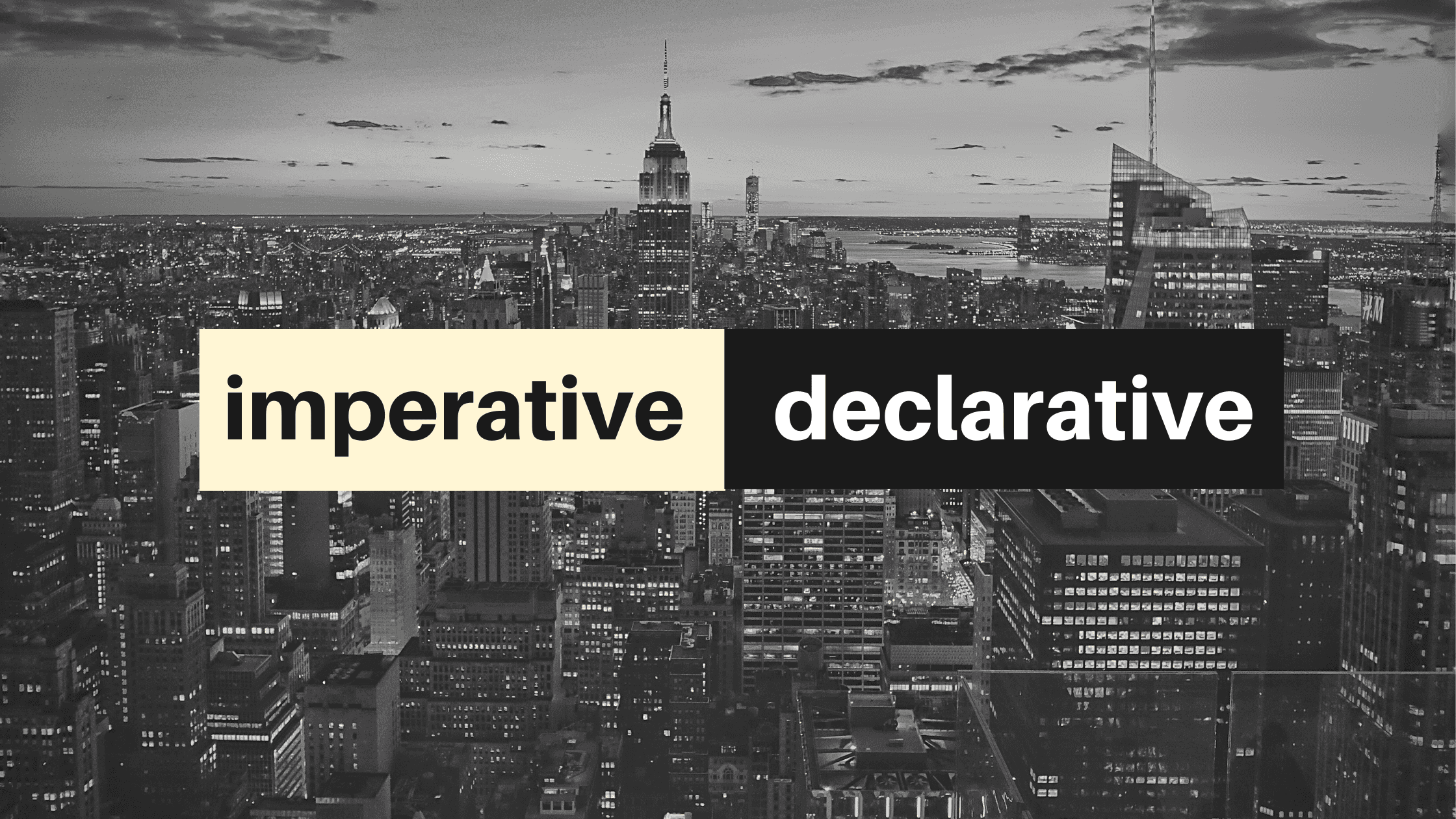
Imperative vs Declarative in React
August 27th, 2019
When you start using React you'll hear a lot about imperative versus declarative code.
The definition
Imperative programming is like how you do something, and declarative programming is more like what you do.
That definition is like trying to answer "What came first, the chicken or the egg?"
Let's clarify what that means...
Consider I'm in my car and I want to keep the temperature somewhere comfortable, maybe that's around 71 degrees. A car has two knobs to reach that state, a knob that controls the temperature and one that controls the air flow.
When it gets too hot or too cold I have to do imperative work. I have to manage these knobs the entire drive. I accumulate this state of 71 degrees over time but I never actually talk about the state.
Now let's consider I get a new car. It doesn't have these knobs for climate control, instead it let's me declare a temperature. Instead of fiddling around with the knob over the course of my drive to get to 71 degrees I just tell the car the state I want to be in. I set the temperature to 71 degrees and it handle the imperative work for me.
React let's you do the same thing with your code. You declare the state and the markup then React does the imperative work of keeping the DOM in sync with your app.
Imperative Code
A lot of JavaScript is imperative code, when JavaScript code is written imperatively, we tell JavaScript exactly what to do and how to do it. Think of it as if we're giving JavaScript commands on exactly what steps it should take
jsconst people = ["Mukesh", "Hera", "Sonali", "Puja", "Sagen", "Sumit"];const excitedPeople = [];for (let i = 0; i < people.length; i++) {excitedPeople[i] = people[i] + "!";}
Declarative Code
With declarative code, we don't code up all of the steps to get us to the end result. Instead, we declare what we want done, and JavaScript will take care of doing it.
jsconst people = ["Mukesh", "Hera", "Sonali", "Puja", "Sagen", "Sumit"];const excitedPeople = people.map((name) => name + "!");
Note
Imperative code instructs JavaScript on how it should perform. With declarative code, we tell JavaScript what we want to be done.
Conclusion
all postsReact is declarative because we write the code that we want, and React is in charge of taking our declared code and performing all of the JavaScript/DOM steps to get us to our desired result.