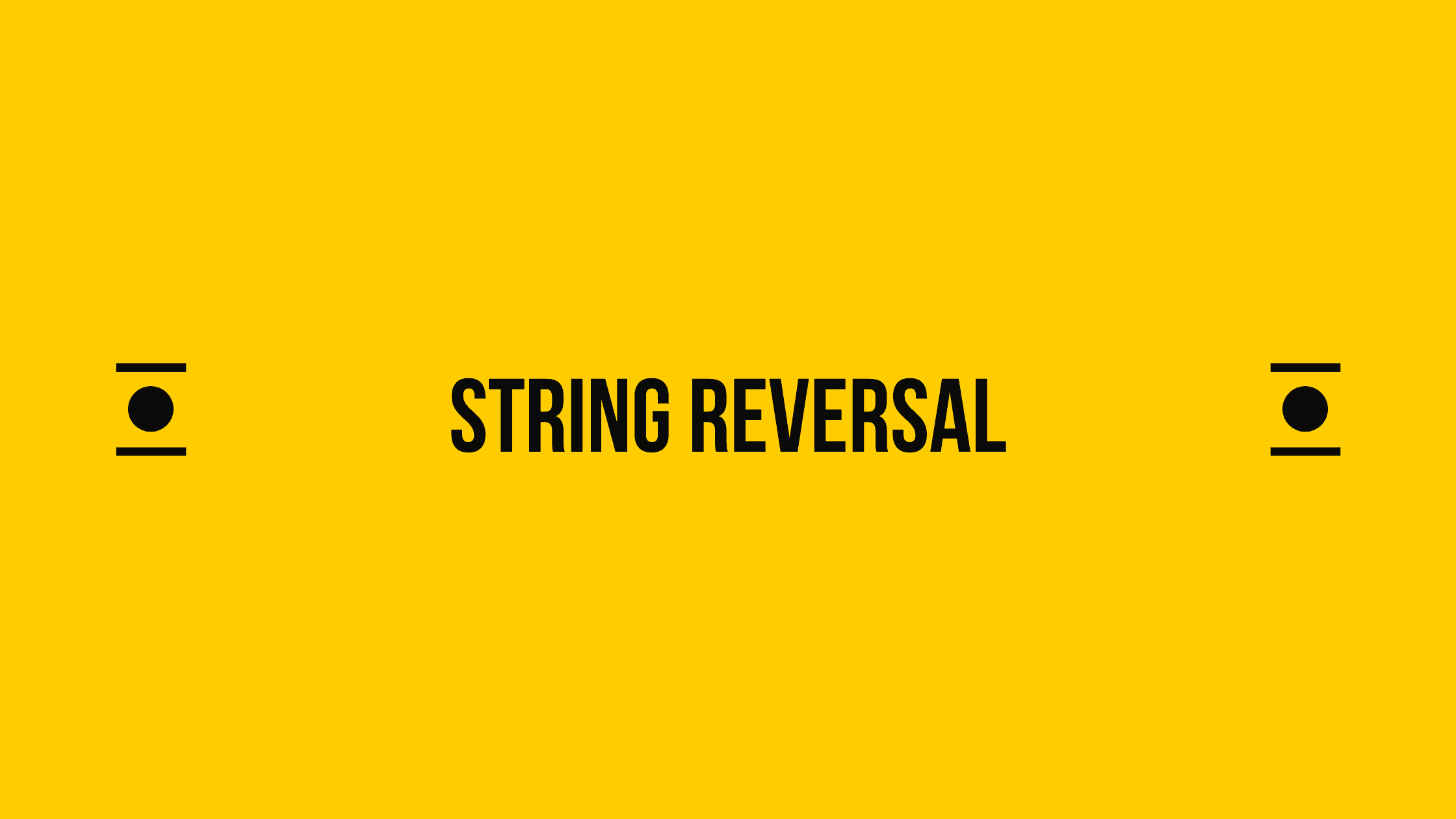
String reversal in Javascript
August 31st, 2019
In this article we're going to walk through some of the possible solution to reverse a given string in JavaScript. This might requires a little bit of knowledge of JavaScript in general.
Problem Statement
Given a string, return a new string with the reversed order of characters.
Now essentially all we really want to do here is take a string that is provided as an argument. Reverse it and then return it from the function. And the last part is really important. Don't forget to return the string that gets reversed.
🎈 Method 1
Now the first solution that we're gonna start off with is by far the easiest one. However it's also not necessarily the most obvious one.
jsfunction reverse(str) {const arr = str.split("");arr.reverse();return arr.join("");}reverse("MajhiRockzZ");
we can do a little bit of code cleanup to make this function a little bit more concise than it is.
jsfunction reverse(str) {return str.split("").reverse().join("");}reverse("MajhiRockzZ");
🎁 Method 2
In the previous method we put together one very straightforward solution for reversing a string. But what if we want the solution without using the reverse helper. obviously if you know this thing exists it really makes the question quite easy and quite straightforward. So in this section we're going to look at an alternative solution that's a little bit more manual work.
jsfunction reverse(str) {let reversed = "";for (let character of str) {reversed = character + reversed;}return reversed;}reverse("sumit");
So this is one possible solution.
🍕 Method 3
We have now put together two possible solutions to the string reversal problem. In addition I'm going to show you one more complicated ways of solving this problem. So in this last solution we are going to use a very complicated little array helper. So let's give this last solution a shot.
jsfunction reverse(str) {return str.split("").reduce((reversed, character) => {return character + reversed;}, "");}reverse("hello");
The last thing that i would probably do here is to simplify some of the syntax a little bit.
jsfunction reverse(str) {return str.split("").reduce((rev, char) => char + rev, "");}reverse("hello");
Conclusion
all postsSo any three of these solutions works 100 percent just fine. And of course, there are other solutions out there that might be better than these three.